Aligning product cards with subgrid
I've always wanted to learn about subgrids, but I never really needed them—until last week. We were presenting a new feature to the stakeholders when suddenly our UX Designer noticed the content alignment was off. The UI was something like this:
Kitchen
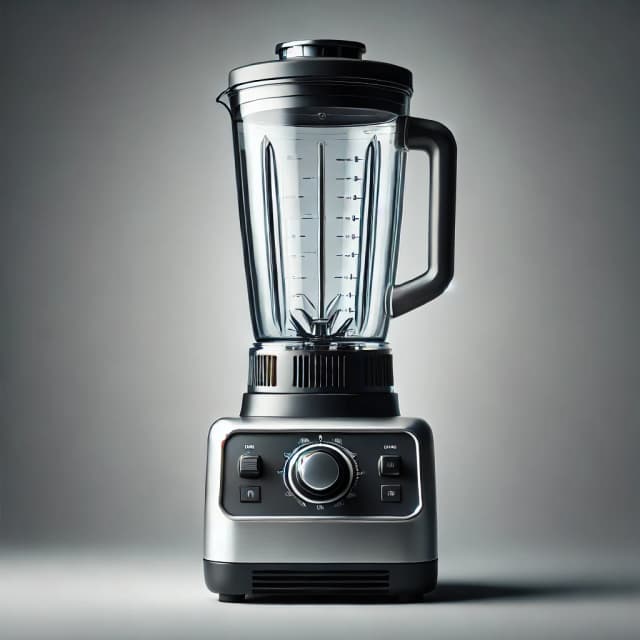
Professional Blender
Blend, mix, and puree with ease using our professional-grade blender. Features a powerful motor, multiple speed settings, and durable stainless steel blades.
Electronics
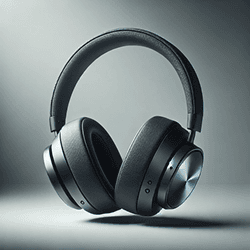
Wireless Noise-Canceling Headphones
Experience immersive sound with our premium wireless noise-canceling headphones.
Wearable Tech and Advanced Fitness Tracking Devices
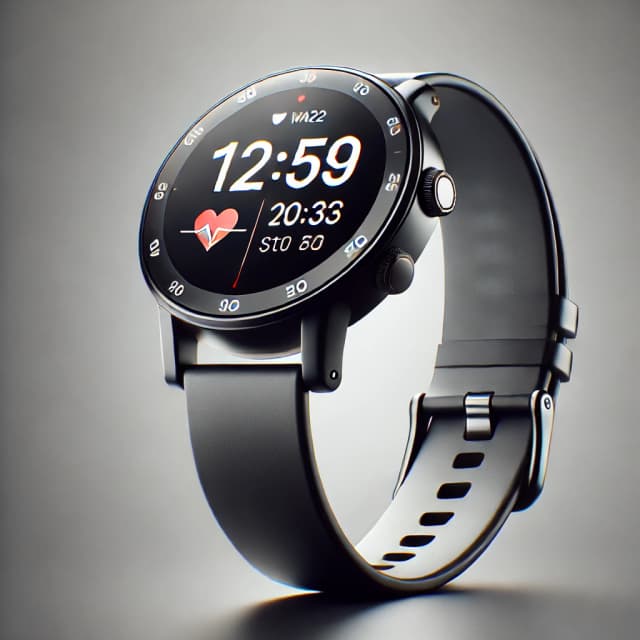
Smartwatch with Fitness Tracking
Stay on top of your health and notifications with our advanced smartwatch.
Ok, the misalignment wasn't so visible as the one in the example, but you get the point. The content from the cards are not aligned with each other due to how dynamic they are. The length of the texts and the amount of promotion labels can vary a lot, causing the misalignments. The UX designer asked if we could improve it by making sure the image and the price were always aligned with each other. This seemed like a perfect use case for subgrids.
What is a subgrid?
Subgrid is a feature of the CSS grid. With CSS grid you can divide a container in columns and rows in order to better layout the content of your web page. Basically, you define a grid for an element and then all its direct children become cells that follow those grid rules. You can also determine the space each cell is going to occupy in the grid. In the example below, we are creating a grid where the first cell spans 2 columns and 1 row, while the other two take up 1 column and 1 row each.
<div class="grid"> <div class="grid-item">1</div> <div class="grid-item">2</div> <div class="grid-item">3</div> </div> <style> .grid { display: grid; /* Define 3 columns with 1 fraction each */ grid-template-columns: repeat(3, 1fr); /* Define 150px as the height of each row */ grid-auto-rows: 150px; /* Spacing between grid items */ gap: 16px; } .grid-item { padding: 16px; } .grid-item:first-child { /* First grid item fills the space of two columns */ grid-column: span 2; background: #fed7aa; } .grid-item:nth-child(2) { background: #d9f99d; } .grid-item:last-child { background: #bae6fd; } </style>
Note: If you want to learn more about CSS grid, I recommend reading the complete css grid guide.
Subgrid is a value you can use for both grid-template-columns and grid-template-rows properties to make a child element inherit the grid's structure from its parent instead of defining a new one. See in the example below how the children of the grid-item are glued with the grid structure from the grid element.
<div class="grid"> <div class="grid-item"> <div>Grid item child</div> <div>Grid item child</div> </div> <div class="grid-item"> <div>Grid item child</div> <div>Grid item child</div> </div> <div class="grid-item"> <div>Grid item child</div> <div>Grid item child</div> </div> </div> <style> .grid { display: grid; /* Define 3 columns with 1 fraction each */ grid-template-columns: repeat(3, 1fr); /* Define 2 rows with 100px each */ grid-auto-rows: 100px 100px; /* Spacing between columns of the grid items */ column-gap: 16px; /* Spacing between the rows of the grid items */ row-gap: 8px; } .grid-item { display: grid; /* Make the grid item to use the grid structure of its parent */ grid-template-rows: subgrid; grid-row: span 2; } .grid-item > div { padding: 16px; } .grid-item:first-child { background: #fed7aa; } .grid-item:nth-child(2) { background: #d9f99d; } .grid-item:last-child { background: #bae6fd; } </style>
That comes handy for the challenge we have, since we need the elements of each card to be aligned with each other.
Let's try it out.
Implementing subgrids to our product cards
Alright, we already know we can use subgrids to solve our issue. For that, we have to set a grid for the product cards container taking into account the rows needed for the content of the product card, and then make each product card a subgrid to inherit its parent grid's structure. But what grid rules do we need to define?
First, we have to identify what are the rows that compose our product card and how they should behave. We identified 3 rows:
- The header: composed by the category and the image
- The content: composed by the title and description
- The footer: composed by the promotion labels and the price
Category
Title
Description
Category
Title
Description
Category
Title
Description
We can use the following markup to implement it:
<div class="product-cards"> <div class="product-card"> <div class="product-card__header"> <span class="product-card__category">Category</span> <div class="product-card__image">Image</div> </div> <div class="product-card__content"> <h2 class="product-card__title">Title</h2> <p class="product-card__description">Description</p> </div> <div class="product-card__footer"> <div class="product-card__promo-labels"> <span class="product-card__promo-label">Promo label 1</span> <span class="product-card__promo-label">Promo label 2</span> </div> <p class="product-card__price">Price</p> </div> </div> </div>
To ensure a proper alignment between cards, the rows should stretch based on the content's length of each product card. For the columns, nothing needs to be done.
Now that we know how many rows we need and how they should behave, it is quite simple. We set the product card grid-template-rows to subgrid and configure it to span 3 rows using the grid-row property. So far, this is the result:
Kitchen
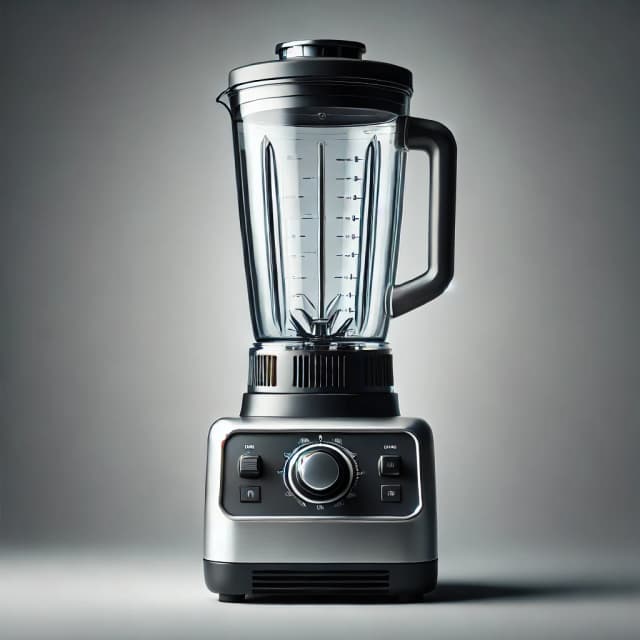
Professional Blender
Blend, mix, and puree with ease using our professional-grade blender. Features a powerful motor, multiple speed settings, and durable stainless steel blades.
Electronics
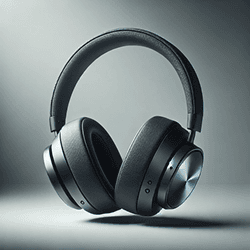
Wireless Noise-Canceling Headphones
Experience immersive sound with our premium wireless noise-canceling headphones.
Wearable Tech and Advanced Fitness Tracking Devices
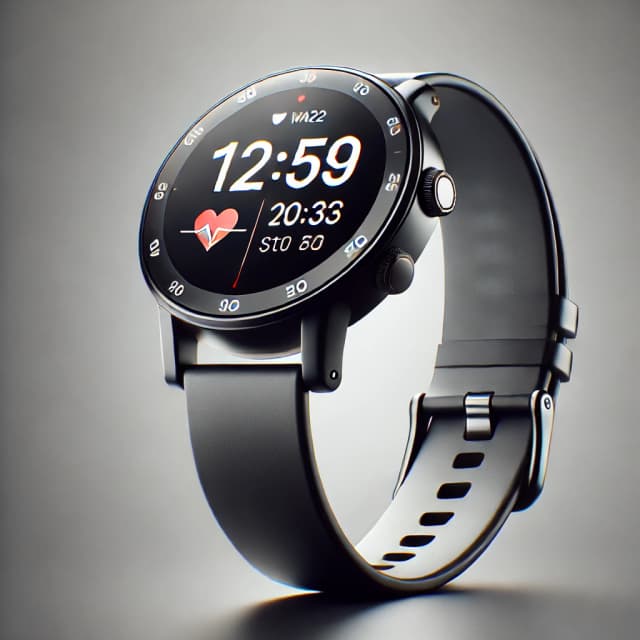
Smartwatch with Fitness Tracking
Stay on top of your health and notifications with our advanced smartwatch.
.product-cards { display: grid; grid-template-columns: repeat(3, 232px); gap: 16px; } .product-card { display: grid; grid-template-rows: subgrid; grid-row: span 3; gap: 16px; }
We still have to make some tweaks to give a better sense of alignment since the row heights may stretch based on content's length, so it is good to ensure the position of the elements are correct.
.product-card__header { /* In order to align the image */ display: grid; } .product-card__content { align-content: start; } .product-card__footer { align-content: end; } .product-card__image { align-self: end; }
And this is the final result:
Kitchen
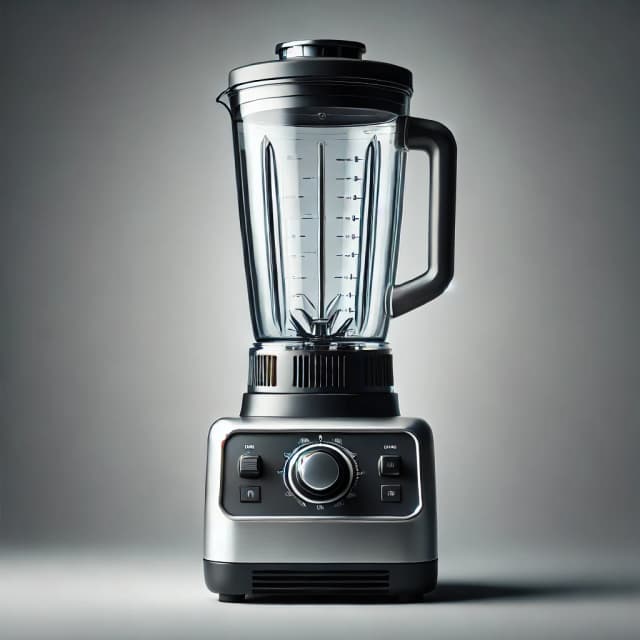
Professional Blender
Blend, mix, and puree with ease using our professional-grade blender. Features a powerful motor, multiple speed settings, and durable stainless steel blades.
Electronics
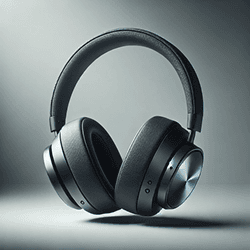
Wireless Noise-Canceling Headphones
Experience immersive sound with our premium wireless noise-canceling headphones.
Wearable Tech and Advanced Fitness Tracking Devices
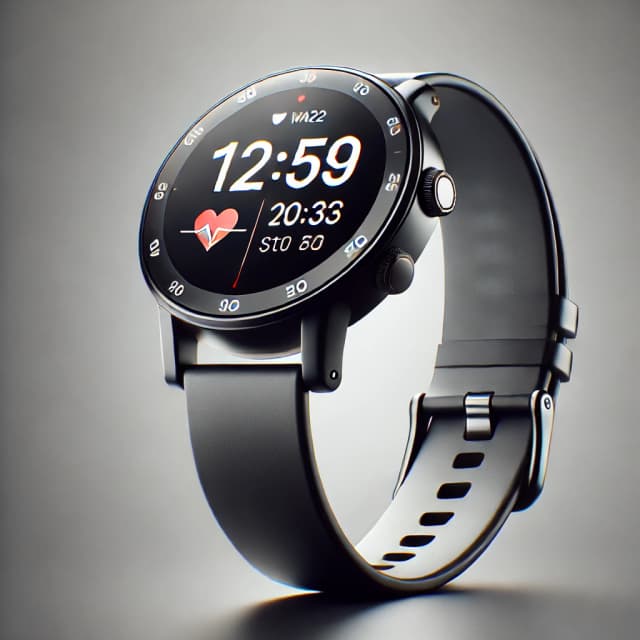
Smartwatch with Fitness Tracking
Stay on top of your health and notifications with our advanced smartwatch.
Once we implemented the adjustments, we checked with the UX designer again, and it was exactly what they had envisioned. Pretty nice, right? So that's the story of how I finally learned—and used—subgrids at work. I hope this real-world example helped you understand subgrids a little better. See you next time.